Chrome拡張でContent ScriptとBackground Pageのメッセージのやり取りをする
以前の記事でやりたいこととして挙げていた、
を、ようやく実装できました。
Chrome拡張「Amazon to Rakuten」は当初下記の流れで作っていました。
2. XMLHttpRequestで取得したURLのHTMLを取ってきて、本のタイトルを正規表現で取得
3. 楽天ブックス総合検索APIに取得したタイトルを投げる
4. 検索結果をPopupに表示。表示されたやつをクリックすると無事楽天ブックスの商品詳細ページにジャンプ
が、この1.と2.の部分が無駄なのとbackgroundPageを使ってみたかったのとで、次のような流れにしました。
2. 取得したタイトルをMessageでbackground.htmlに送信
3. background.html上で楽天ブックス総合検索APIに、Content Scriptから受信した商品タイトルを投げて返ってきた結果を処理し、popup.htmlに表示したい内容を変数に格納
4. popup.htmlには3.で格納したbackground.html内の変数の中身を表示するスクリプトを書いておく
こうすることで、amazonの商品ページを表示した瞬間に裏側でDOM解析&API検索を行い、アイコンをクリックしたらその処理結果を表示するだけになるので、アイコンクリック後の待ち時間がほぼなくなります。今回も色んなところで詰まりまくった。
1. Content Scriptで商品タイトルを引っ張ってくる
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
/* manifest.json */
{
・・・
"background_page": "background.html",
"content_scripts": [
{
"matches": [
"http://www.amazon.co.jp/*",
"http://www.amazon.com/*"
],
"js": ["script.js"],
"run_at": "document_end"
}
],
"browser_action": {
"default_icon": "icon.png",
"popup": "popup.html"
},
・・・
}
まずはここから。Content Scriptを使うには、manifest.jsonに上記のように記述する必要があります。
"matches"はContent Scriptを動かすサイトの条件を設定する項目。
"js"は動かしたいJavaScriptファイルのファイル名。
"run_at"はどのタイミングでスクリプトを実行するかを決める項目。今回はDOM構築完了時にスクリプトを動かす"document_end"にしてます。
他にも何個かオプションがあります。詳細はこちら。
1
2
3
4
/* script.js */
title = document.getElementById('btAsinTitle').firstChild.nodeValue;
title.match(/([^\(]*)/);
title = RegExp.$1;
こうすると、amazonの詳細ページでDOM構築が終わった瞬間script.jsが実行されて、titleの中に商品タイトルが入るって寸法です。
これをそのままAPIに投げたいところですが、Content ScriptではXMLHttpRequestが使えないそうなので、background.htmlに商品タイトルを送ってやる必要があります。
2. Content Scriptからbackground.htmlにメッセージを送信
1
2
3
4
5
6
7
8
9
10
11
12
/* script.js */
title = document.getElementById('btAsinTitle').firstChild.nodeValue;
title.match(/([^\(]*)/);
title = RegExp.$1;
var port = chrome.extension.connect({name: "AtoR"});
port.postMessage({title: title,status:"start"});
port.onMessage.addListener(function(msg) {
if (msg.status == "loading"){
port.postMessage({status: "loading"});
}
});
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
/* background.html */
chrome.extension.onConnect.addListener(function(port) {
console.assert(port.name == "AtoR");
port.onMessage.addListener(function(msg) {
if(msg.status == "start"){
//楽天APIから商品を検索
query = "http://api.rakuten.co.jp/rws/3.0/json?" +
"developerId=" + devId +
"&affiliateId=" + afiId +
"&operation=" + opr +
"&version=" + ver+
"&keyword=" + encodeURI(msg.title);</p>
api.open("GET",query,true);
api.onreadystatechange = sourceGet(port);
api.send(null);
}
else{
sourceGet(port);
}
});
});
function sourceGet(port){
console.log("sourceGet");
if (api.readyState == 4 && api.status == 200){
//APIからのレスポンスがきちんと返ってきた時の処理
・・・
}
else{
if (loading === false){
loading = true;
content = '<img src="loading.gif" />';
}
port.postMessage({status: "loading"});
}
}
例によって詳細はChromeAPIのメッセージのページを見てください。
今回はXMLHttpRequestを使うせいか単発通信ではうまくいかなかったので、永続通信で下記の流れでやり取りさせてます。
↓
background.htmlがstartを受け取ったら楽天APIにタイトルを投げてsourceGetを実行。通信中の場合はstatus:loadingをContentScriptに返す
↓
ContentScriptにstatus:loadingが返ってきたら再びstatus:loadingを投げ返し、background.htmlにお伺いを立てる
↓
background.htmlで再びsourceGetを実行。APIからの返事がまだならもっかいstatus:loadingを投げ返す、返事が来てたら処理終了
ってな具合です。
3. popup.htmlに表示したい内容を変数に格納
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
/* background.html */
function sourceGet(port){
console.log("sourceGet");
if (api.readyState == 4 && api.status == 200){
response = eval('[' + api.responseText + ']')[0];</p>
if(response['Header']['Status'] == 'Success'){
items = response['Body']['BooksTotalSearch']['Items']['Item'];</p>
//商品データを1つずつhtmlに出力
content = '<table width="300">';
for(i=0;i<items.length;i++){
content += '<tr><td><a href="'+items[i]['affiliateUrl']+'"><img src="'+items[i]['mediumImageUrl']+'" /></a></td>' +
'<td style="width:200px;vertical-align:top;">タイトル:' +
'<a href="'+items[i]['affiliateUrl']+'" target="_blank">' + items[i]['title'] + '</a><br />' +
'著者:' + items[i]['author'] + '<br />' +
'価格(税込):' + setComma(items[i]['itemPrice']) + '円<br />' +
'ポイント:'+Math.floor(items[i]['itemPrice']/1.05/100)+'ポイント</td></tr>';
}
content = content+'</table>';
}
else if(response['Header']['Status'] == 'NotFound'){
content = '見つかりませんでした。';
}
else{
content = 'エラーが発生しました。';
}
}
else if (api.readyState == 4){
content = "接続に失敗しました。";
}
else{
if (loading === false){
//ロード中画像を表示
loading = true;
content = '<img src="loading.gif" />';
}
port.postMessage({status: "loading"});
}
}
実際のsourceGetはこんな感じです。汚い。
ポップアップに表示したいものは全部変数contentに格納して、後から引っ張ってきます。
4. background.htmlのcontentに格納した内容をpopup.htmlに表示
1
2
3
4
5
6
7
8
9
10
11
12
13
/* popup.html */
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
</head>
<body>
<div id="content"></div>
<script>
bg = chrome.extension.getBackgroundPage();
document.getElementById('content').innerHTML = bg.content;
</script>
</body>
</html>
なんと、たったの2行で、backgound.html上の変数をpopup.htmlでも使うことができてしまいます。
逆だとちょっとめんどいです。詳細はこちら。
ちなみに、script.jsは外から呼べなかったです。なぜ。
思わぬ落とし穴
これでようやく思ったとおりの挙動になって万々歳!と思った矢先、思わぬ落とし穴が。
このbackground.htmlはタブを何個開いてても共通で使われるので、例えば次のような場合。
↓
商品2が読み込まれた瞬間background.htmlに商品2のデータが格納される
↓
商品1のタブに戻って拡張のアイコンをクリック
↓
background.htmlには商品2のデータが格納されたままなので、そっちが表示されてしまう
というわけで、タブを切り替える度にタイトルを取得してAPIを叩き直さんとあかんことに気づく。
ですが予想通りタブ切り替えイベントは用意されていたので、ささっと対応しました。
1
2
3
4
5
6
7
8
9
10
11
/* background.html */
・・・
//タブが変更された時の処理
chrome.tabs.onSelectionChanged.addListener(function(tabid){
chrome.tabs.getSelected(null, function(tab) {
chrome.tabs.sendRequest(tab.id, {status: "changed"}, function(response) {});
});
});
・・・
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
/* script.js */
AtoR();
chrome.extension.onRequest.addListener(
function(request, sender, sendResponse) {
AtoR();
}
);
function AtoR(){
title = document.getElementById('btAsinTitle').firstChild.nodeValue;
title.match(/([^\(]*)/);
title = RegExp.$1;
var port = chrome.extension.connect({name: "AtoR"});
port.postMessage({title: title,status:"start"});
port.onMessage.addListener(function(msg) {
if (msg.status == "loading"){
port.postMessage({status: "loading"});
}
});
}
chrome.tabs.onSelectionChangedがscript.js側で使えればよかったんですが、ContentScript上では使えませんって怒られたのでbackground.htmlに記述して例によってメッセージで送信。
chrome.extension.onRequest.addListenerの中がメッセージ受信時、つまりタブが切り替わった時に実行されるアクションです。
そんなこんなで
あれやこれやと試行錯誤を繰り返し、なんとかやりたいことを1つ実装できました。今後もちょくちょくアップデートしていく予定です。
Chrome拡張はインストールしたらソースコード全部見られるので、興味ある方はぜひ。
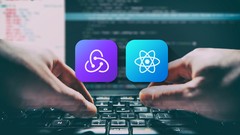
「最短で学ぶReactとReduxの基礎から実践まで」10%OFFクーポン
UdemyでReactとReduxの動画講座を公開しています。
このブログの読者限定クーポンを使って、基礎から実践までを学びましょう。
「最短で学ぶReactとReduxの基礎から実践まで」10%OFFクーポン
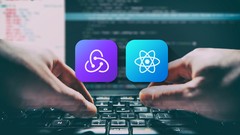
UdemyでReactとReduxの動画講座を公開しています。
このブログの読者限定クーポンを使って、基礎から実践までを学びましょう。